In Da Range
Published on Dec 10, 2019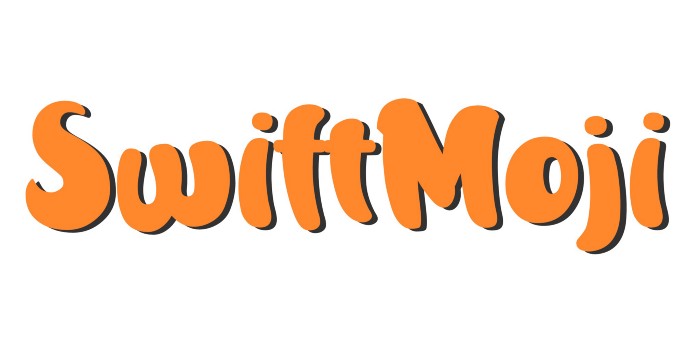
In Da Range — SwiftMoji Entry #16
Swift provides the pattern matching operator (~=) for matching cases in switch statements. If the operator is overloaded, the specified type of the lhs (left-hand side) can be compared against various values of the rhs in a switch statement. Although the ~= operator is hidden under the switch statement’s syntax, it can still be used throughout code directly. In the code example, a musical note is checked to be within the Range of notes of a musician using the ~= operator.
import Foundation
struct Note: Comparable {
enum NoteLetter: Int {
case A, Bb, B, C, Db, D, Eb, E, F, Gb, G, Ab
}
let letter: NoteLetter
let octave: Int
init(_ letter: NoteLetter, _ octave: Int) {
self.letter = letter
self.octave = octave
}
static func < (lhs: Note, rhs: Note) -> Bool {
if lhs.octave != rhs.octave {
return lhs.octave < rhs.octave
}
return lhs.letter.rawValue < lhs.letter.rawValue
}
}
struct Musician {
let instrument: String
let range: ClosedRange<Note>
}
var 👨🏻🦲 = Musician(instrument: "🎺", range: Note(.B, 4)...Note(.Gb, 6))
if 👨🏻🦲.range ~= Note(.Gb, 3) {
print("Awesome, you are a professional!")
} else {
print("You must practice more.")
}
Tagged with: